x and y being two signals, either 0 (false) or 1 (true)
basic boolean operations (AND, OR, NOT)
// X AND Y: only both together trigger (intersection) x * y // X OR Y: either one triggers (union) x + y > 0 // NOT X: triggers not (complement) 1 - x
some derived boolean operations
// X XOR Y: either one triggers, but not both x + y % 2 // NAND: not both trigger 1 - (x * y) // NOR: not any one triggers, only if none are on. (1-x) * (1-y)
an example in SuperCollider
server side
( { var x, y; x = LFPulse.ar(1200, 0, 0.2); y = LFPulse.ar(1600, 0, 0.4); [ x, y, x * y, x + y > 0, 1 - x, x + y % 2, 1 - (x * y) ] * 0.5 }.scope; )
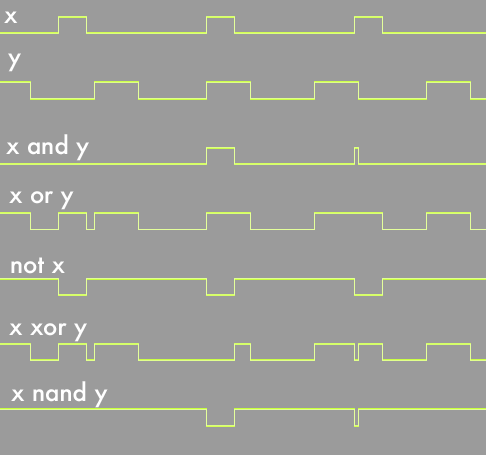
in sc, also "if" can be used as a signal flip-flop (see "if"-helpfile)
Note that in SuperCollider, a trig input usually means any change of a signal from nonpositive to positive.
This means that an LFPulse will always trigger when "opening", not when "closing".
client side
The above operations can be used on signals directly. On the language side (sc-lang) of course booleans can be used directly:
and(x, y) // synonyms: x && y, x and: y or(x, y) // synonyms: x || y, x or: y not(x) xor(x, y) // synonyms: x xor: y not(and(x, y))
in order to convert them into a binary number, the messages True-binaryValue and False-binaryValue can be used:
x = true; x.binaryValue; // returns 1
bitwise boolean operations
see for example the picture here& bitwise AND (bitAnd) | bitwise OR (bitOr) bitXor bitwise XOR
boolean valves
// boolean valves p = ProxySpace.push(s.boot); ~rate = 1.2; ~x = { LFPulse.kr(~rate.kr, 0, 0.3) }; ~y = { LFPulse.kr(~rate.kr * 4/3, 0, 0.3) }; ~out.play; // and ( ~out = { var a = ~x.kr, b = ~y.kr; SinOsc.ar( [ a * 300 + 250, b * 400 + 300, (a * b) * 800 + 400 ] ).sum * 0.1 } ) // or ( ~out = { var a = ~x.kr, b = ~y.kr; SinOsc.ar( [ a * 300 + 250, b * 400 + 300, (a + b > 0) * 800 + 400 ] ).sum * 0.1 } ) // not x ( ~out = { var a = ~x.kr, b = ~y.kr; SinOsc.ar( [ a * 300 + 250, b * 400 + 300, (1 - a) * 800 + 400 ] ).sum * 0.1 } ) // xor ( ~out = { var a = ~x.kr, b = ~y.kr; SinOsc.ar( [ a * 300 + 250, b * 400 + 300, (a + b % 2) * 800 + 400 ] ).sum * 0.1 } ) // nand ( ~out = { var a = ~x.kr, b = ~y.kr; SinOsc.ar( [ a * 300 + 250, b * 400 + 300, (1 - (a + b)) * 800 + 400 ] ).sum * 0.1 } ) // monitor the triggers ~trig.play; ~trig = { Ringz.ar(~x.ar, 1000 * [1, 1.3], 0.1) * 0.3 }; ~trig = { Ringz.ar(~y.ar, 800 * [1, 1.3], 0.1) * 0.3 }; ~trig.stop; // end the whole p.end(1); p.clear;